Create an Azure Key Vault with RBAC role assignments using Terraform
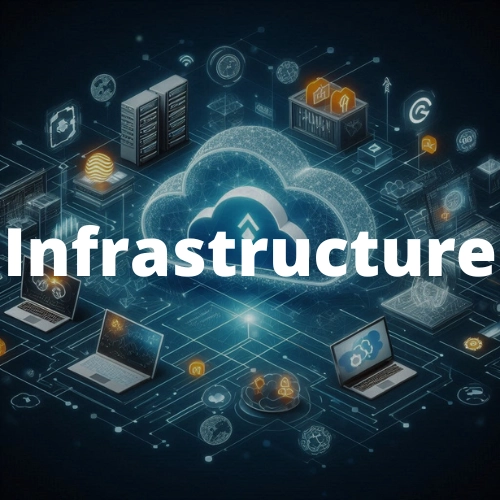
Azure Key Vault is a Microsoft Azure resource that securely stores and manages sensitive data, including secrets, encryption keys, and certificates, it features soft deletion of secrets, renovation of certificates, and security features including Role-Based Access Control (RBAC) and private network access.
In this post, I will show you how to use Terraform to create an Azure Key Vault and populate it adding some secrets. Additionally, I will show you how to use Terraform to assign a user a role so that they can create secrets in Azure Key Vault.
Create an Azure Key Vault using Terraform
What do you need
Your
subscription_id
, this is a GUID and you can get it using one of the following ways:Using Azure CLI:
az account subscription list
Using the Azure Portal.
Your
tenant_id
, this is a GUID and you can get it using one of the following ways:Using Azure CLI:
az account tenant list
Using the Azure Portal in the column
Directory ID
.
Terraform files
main.tf
terraform {
required_providers {
azurerm: {
source : "hashicorp/azurerm"
version: "4.11.0"
}
}
}
provider "azurerm" {
# Configuration options
features {
key_vault {
purge_soft_delete_on_destroy : true
recover_soft_deleted_key_vaults: true
}
}
subscription_id: "a838fdd3-56b8-4508-93db-9611367b3aee" # Put your 'subscription_id' here
tenant_id: "c4421ca3-5bd8-472b-99c4-6b231540eac1" # Put your 'tenant_id' here
}
data "azurerm_client_config" "current" {}
In this file you declare the Terraform provider.
keyvault.tf
resource "azurerm_resource_group" "secrets_rg" {
name : "secrets"
location: "westus3"
}
resource "azurerm_key_vault" "secrets_keyvault" {
tenant_id : data.azurerm_client_config.current.tenant_id
resource_group_name: azurerm_resource_group.secrets_rg.name
name : "secrets"
location: azurerm_resource_group.secrets_rg.location
enable_rbac_authorization : true
enabled_for_deployment : false
enabled_for_disk_encryption : false
enabled_for_template_deployment: true
public_network_access_enabled : false
purge_protection_enabled : true
soft_delete_retention_days : 90
sku_name: "standard"
}
You can customize the Azure Key Vault in this file.
secrets.tf
resource "azurerm_key_vault_secret" "db_development" {
name : "DbConnectionStringDevelopment"
value : "mysql://root:password123@db.example.com:3306/mydatabase"
key_vault_id: azurerm_key_vault.secrets_keyvault.id
}
This file will store non-sensitive secrets, such as secrets for development or integration environments.
Role assignment using Terraform
Now we are assigning a user a role to access and manage secrets in the Azure Key Vault previously created.
Azure RBAC has some built-in roles that can be assigned to a user or group, allowing granular control over access to Azure Key Vault.
What do you need
The
principal_id
of the user or group (also known asObject ID
), this is a GUID and you can get it using one of the following ways:Using Azure CLI:
az ad user list --query "[].{Id:id, Name: displayName, Email: mail}" --output table
Using the Azure Portal.
The
role_definition_id
of the role that you want to assign, this is a GUID and you can get it using one of the following ways:Using Azure CLI:
az role definition list --query "[?contains(roleName, 'Key Vault')].{Name:name, Description: description}" --output table
Using the Microsoft Docs in the column
ID
.
Terraform files
rbac.tf
resource "azurerm_role_assignment" "keyvault_secrets_officer_john" {
scope: azurerm_key_vault.secrets_keyvault.id
principal_id : "8c4ab003-5dcd-49e2-86df-8f96b1f46a0d" # Put your 'principal_id' here
role_definition_id: "b86a8fe4-44ce-4948-aee5-eccb2c155cd7" # Put your 'role_definition_id' here
}
References
Categories
Automation Development tools Infrastructure Kubernetes Programming guide Software architectureTags
Recent Posts