Restart Kubernetes pods following a schedule using Helm
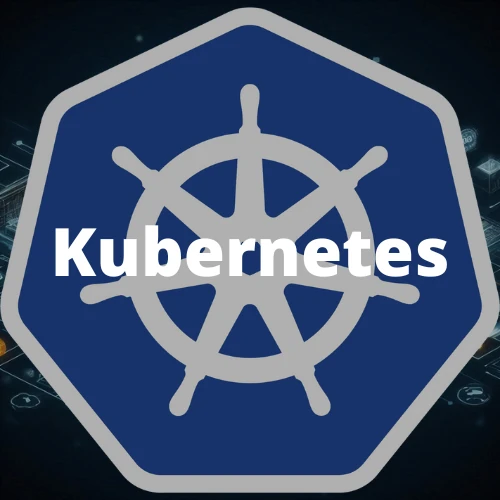
In my previous post I showed you how to restart Kubernetes pods following a schedule using Kubectl. This time, I will show you how to do the same using Helm.
Helm is a package manager for Kubernetes that simplifies application deployment and management by packing pre-configured Kubernetes resources.
Practical case
Let’s continue using the practical case of the previous post, imagine that we want to restart all the pods of this Kubernetes Deployment:
apiVersion: apps/v1
kind: Deployment
metadata:
name: hello-world
namespace: apps
spec:
replicas: 5
selector:
matchLabels:
app: app1
template:
metadata:
labels:
app: app1
spec:
containers:
- image: crccheck/hello-world
name: hello-world
It uses the label “app”, so, the Kubectl command to restart all the pods of this Kubernetes Deployment is:
kubectl delete -n apps pods --selector=app="app1"
As we remember from the previous post, we need to:
- Create an identity for the pod for which we will use a Kubernetes ServiceAccount.
- Create a set of permissions for that identity for which we will use a Kubernetes Role.
- Assign the set of permissions to the identity, for which we will use a Kubernetes RoleBinding.
- Deploy a Kubernetes CronJob with the identity previously mentioned.
Process
Let’s create a new Helm chart:
helm create demo
Delete some of the pre-created files and use this template:
├── charts
├── templates
│ ├── ServiceAccount.yaml
│ ├── Role.yaml
│ ├── RoleBinding.yaml
│ └── CronJob.yaml
├── Chart.yaml
└── values.yaml
Define variables (values.yaml)
# The name of the app from which we will delete its pods.
appName: 'app1'
# The namespace of the app from which we will delete its pods.
appNamespace: 'apps'
# The name that the ServiceAccount will have.
serviceAccountName: 'app1-pod-deleter'
# The name that the Role will have.
roleName: 'delete-pods'
Create the ServiceAccount (templates/ServiceAccount.yaml)
apiVersion: v1
kind: ServiceAccount
metadata:
name: '{{ .Values.serviceAccountName }}'
namespace: '{{ .Values.appNamespace }}'
Create the Role (templates/Role.yaml)
- The target resource is pods.
- The necessary permissions/verbs are:
list
anddelete
.
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
name: '{{ .Values.roleName }}'
namespace: '{{ .Values.appNamespace }}'
rules:
- apiGroups:
- ''
resources:
- pods
verbs:
- list
- delete
Create the RoleBinding (templates/RoleBinding.yaml)
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: '{{ .Values.roleName }}-{{ .Values.serviceAccountName }}-binding'
namespace: '{{ .Values.appNamespace }}'
roleRef:
apiGroup: rbac.authorization.k8s.io
kind: Role
name: '{{ .Values.roleName }}'
subjects:
- kind: ServiceAccount
name: '{{ .Values.serviceAccountName }}'
namespace: '{{ .Values.appNamespace }}'
Deploy the CronJob (templates/CronJob.yaml)
- The cron schedule expressions “0 0 * * *” means to execute it everyday at 00:00.
apiVersion: batch/v1
kind: CronJob
metadata:
name: 'delete-{{ .Values.appName }}-pods'
namespace: '{{ .Values.appNamespace }}'
spec:
jobTemplate:
metadata:
name: 'delete-{{ .Values.appName }}-pods'
spec:
template:
spec:
serviceAccountName: '{{ .Values.serviceAccountName }}'
containers:
- command:
- kubectl
- delete
- pods
- --selector=app={{ .Values.appName }}
image: bitnami/kubectl:1.31.3
name: 'delete-{{ .Values.appName }}-pods'
resources: {}
restartPolicy: OnFailure
schedule: '0 0 * * *'
Categories
Automation scripting Development tools Front end web development Infrastructure Kubernetes Programming guide Security Software architectureTags
Recent Posts
Restart Kubernetes pods following a schedule using Helm
Restart Kubernetes pods following a schedule using Kubectl
Create an Azure Key Vault with RBAC role assignments using Terraform
Get the download url of the latest GitHub release using Bash
Get the download url of the latest GitHub release using PowerShell