Design Patterns - Static Factory Method Pattern
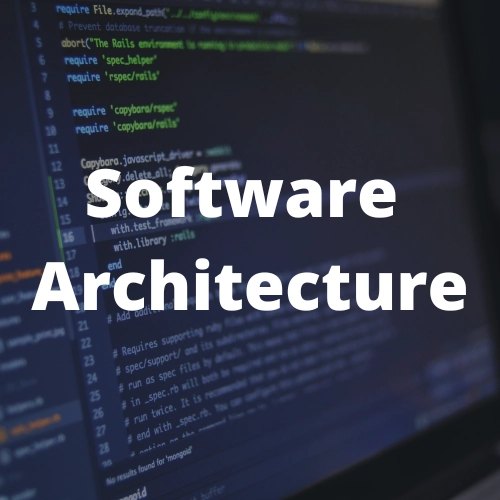
The static factory method is a creational design pattern to create instances of a class, it works like the factory method, but unlike this one, the static factory method just consists of a static method within the class.
This creational design pattern is especially useful in certain scenarios, for example, when the constructor is private.
Examples
Categories
Automation scripting Development tools Front end web development Infrastructure Kubernetes Programming guide Security Software architectureTags
Recent Posts
Restart Kubernetes pods following a schedule using Helm
Restart Kubernetes pods following a schedule using Kubectl
Create an Azure Key Vault with RBAC role assignments using Terraform
Get the download url of the latest GitHub release using Bash
Get the download url of the latest GitHub release using PowerShell